Consider adding very large numbers. The largest magnitude of integers is limited, so we are not able to add 18,274,364,583,929,273,748,459,595,684,373 and 8,129,498,165,026,350,236, since integer variables cannot hold such large values, let alone their sum. The problem can be solved if we treat these numbers as strings of numerals, store the numbers corresponding to these numerals on 2 stacks, and then perform addition by popping numbers from the stacks. The following figure shows an example of application. In this example, numbers 592 and 3784 are added.
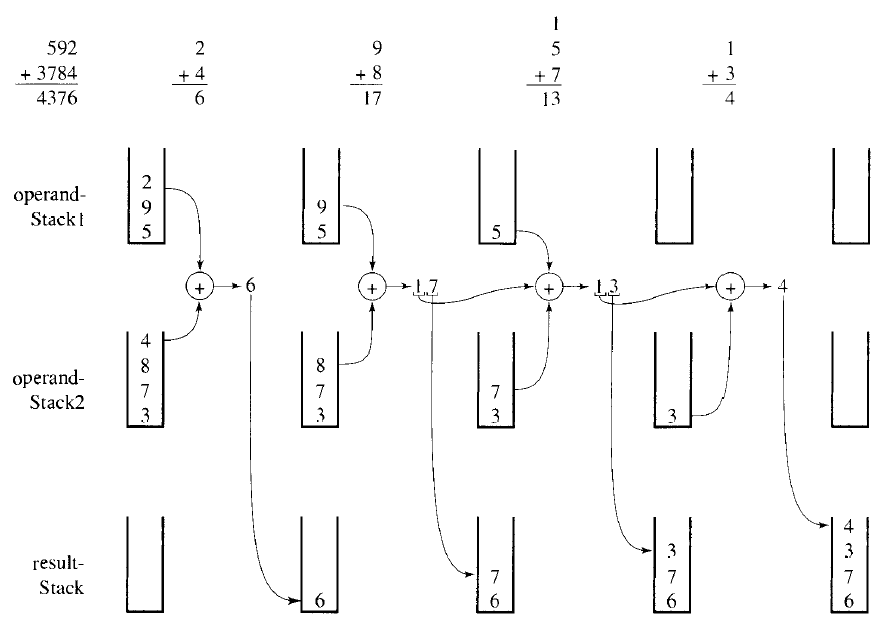
Difficulty level

Video recording
This exercise is mostly suitable for students
void sum(char str1[], char str2[], char str3[])
{
int result=0, i;
element e1=0, e2=0;
stack s1=CreateStack(), s2=CreateStack(), s3=CreateStack();
for(i=0;str1[i];i++)
Push(&s1,str1[i]-'0');
for(i=0;str2[i];i++)
Push(&s2,str2[i]-'0');
while(!isEmptyStack(s1) || !isEmptyStack(s2))
{
if(Top(s1,&e1))
Pop(&s1);
if(Top(s2,&e2))
Pop(&s2);
result += e1+e2;
Push(&s3,result%10);
result /=10;
e1=0, e2=0;
}
if(result)
Push(&s3,result);
i=0;
while(Top(s3,&e1))
{
Pop(&s3);
str3[i++]=e1+'0';
}
str3[i]='\0';
}
Back to the list of exercises
Looking for a more challenging exercise, try this one !!
