Write a program that reads a string \(\texttt{STR}\) from the keyboard and then adds the reverse of \(\texttt{STR}\) to its end (i.e. \(\texttt{STR}\) should contain the original string and its reverse).
You may assume that the size of the string \(\texttt{STR}\) is big enough to hold the string and its reverse.
The usage of any function of the \(\texttt{string.h}\) library is not allowed.
Running example:
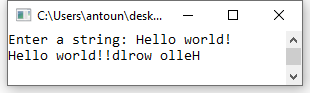
Difficulty level

This exercise is mostly suitable for students
#include <stdio.h>
void main()
{
char STR[100];
int i , j;
printf("Enter a string: ");
gets(STR);
i = 0;
// loop until the \0
while (STR[i]) // go to the end of STR
i++;
// j goes backward from i-1 till 0
j = i - 1;
while (j >= 0) // copy STR to the end of STR
{
STR[i] = STR[j];
i++;
j--;
}
// end of string
STR[i] = '\0';
puts(STR);
}
Back to the list of exercises
Looking for a more challenging exercise, try this one !!
