Write a function that prints the level order data in reverse order. For example, the output for the below tree should be: 0 9 11 8 15 7 35 5
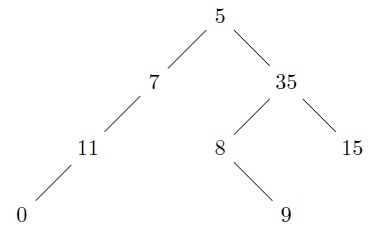
Difficulty level

This exercise is mostly suitable for students
void reversrorder(Btree B)
{
queue q;
stack s;
Btree temp;
if(B)
{
s=CreateStack();
q=CreateQueue();
EnQueue(&q,B);
while(Front(q,&temp))
{
DeQueue(&q);
if(temp->right)
EnQueue(&q,temp->right);
if(temp->left)
EnQueue(&q,temp->left);
Push(&s,temp);
}
while(Top(s,&temp))
{
Pop(&s);
printf("%d ",temp->data);
}
}
}
Back to the list of exercises
Looking for a more challenging exercise, try this one !!
