Write a program that asks the user to enter positive integer values. The program terminates when the user enters a negative value by displaying on the screen the average value and the second distinct maximum of the list of the positive even integers entered by the user.
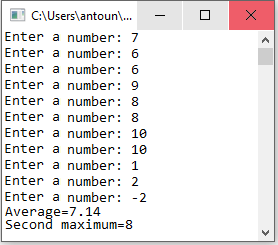
Difficulty level

Video recording
This exercise is mostly suitable for students
#include<stdio.h>
#include<conio.h>
void main()
{
int nb, count = 0, sum = 0, max1 = -1, max2 = -1;
while (1) {
printf("Enter an even number: ");
scanf("%d", &nb);
// order is important
if (nb < 0) break;
if (nb % 2 != 0) continue;
count++;
sum += nb;
if (nb > max1)
{
max2 = max1;
max1 = nb;
}
else
if (nb!=max1 && nb > max2)
{
max2 = nb;
}
}
printf("Second maximum=%d\n", max2);
printf("Average=%.2lf\n", sum*1.0 / count);
getch();
}
#include<stdio.h>
#include<conio.h>
void main()
{
int nb, count = 0, sum = 0, max1 = -1, max2 = -1;
do {
do {
printf("Enter a number: ");
scanf("%d", &nb);
} while (nb > 0 && nb % 2 != 0);
if (nb >= 0) {
count++;
sum += nb;
if (nb > max1)
{
max2 = max1;
max1 = nb;
}
else
if (nb!=max1 && nb > max2)
{
max2 = nb;
}
}
} while (nb>=0);
printf("Average=%.2lf\n", sum*1.0 / count);
printf("Second maximum=%d\n", max2);
getch();
}
Back to the list of exercises
Looking for a more challenging exercise, try this one !!
