Write a function that displays the elements of a binary tree located at odd left to right and top to bottom. The root of the tree is assumed to be at level 0.
Example:
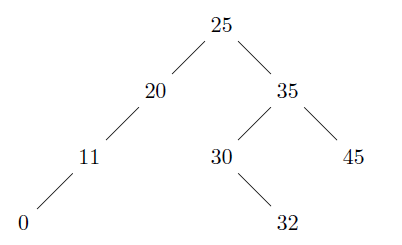
Expected result: 25 11 30 45
Difficulty level

This exercise is mostly suitable for students
void print_odd(Btree B)
{
queue q;
element e;
if(B)
{
q=CreateQueue();
EnQueue(&q,(element){B,0});
while(Front(q,&e) )
{
DeQueue(&q);
if(e.level%2)
printf("%d ",e.B->data);
if(e.B->left)
EnQueue(&q,(element){e.B->left,e.level+1});
f(e.B->right)
EnQueue(&q,(element){e.B->right,e.level+1});
}
}
}
Back to the list of exercises
Looking for a more challenging exercise, try this one !!
