Given a parent array Parent, where Parent[i] indicates the parent of ith node in the tree (assume parent of root node is indicated with –1). Write a fucntion that finds the height or depth of the tree.
Example: If Parent is the following
$ \begin{array}{l}
\begin{array}{|c|c|c|c|c|c|c|c|c|c|}\hline-1&0&1&6&6&0&0&2&7&0\\\hline \end{array} \\ \begin{array}{ c c c c c c c c c c } \ \ \ \ \ 0 & 1 & 2 & 3 & 4 & 5 & 6 & 7& 8 & 9\\ \end{array} \end{array}$
and its corresponding tree is:
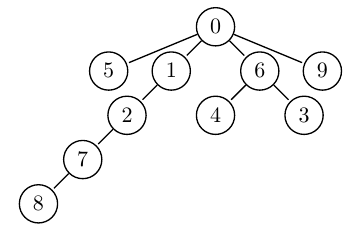
thr height would be equal to 4.
Difficulty level

This exercise is mostly suitable for students
int depthGeneric(int parent[], int size)
{
int i, j;
int currentdepth, maxdepth;
maxdepth = 0;
for(i=0; i<size;i++)
{
currentdepth=0;
j=i;
while(parent[j]!=-1)
{
currentdepth++;
j=parent[j];
}
if(currentdepth>maxdepth)
maxdepth=currentdepth;
}
return maxdepth;
}
Back to the list of exercises
Looking for a more challenging exercise, try this one !!
