Write a program that:
- reads the effective dimension \(\texttt{N}\) of an array (maximum dimension: 50 components);
- then fills two arrays \(\texttt{A}\) and \(\texttt{B}\) each with \(\texttt{N}\) integers entered on the keyboard;
- then fills a third array \(\texttt{C}\) by the inverse summation of \(\texttt{A}\) and \(\texttt{B}\);
- then displays array \(\texttt{C}\).
PS: the inverse summation can be calculated for each element of the array as illustrated below:
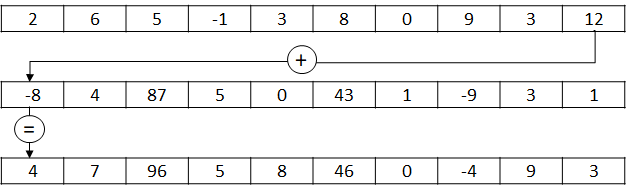
where 12 (last element of the first array) + -8 (first element of the second array) = 4
Running example:
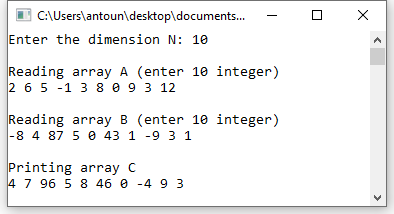
Difficulty level

Video recording
This exercise is mostly suitable for students
#include<stdio.h>
#define SIZE 50
void main()
{
int N;
int A[SIZE], B[SIZE],C[SIZE];
int i, j;
// reading the dimension
do {
printf("Enter the dimension N: ");
scanf("%d", &N);
} while (N<0 || N>SIZE);
printf("\nReading array A (enter %d integer)\n",N);
for (i = 0; i < N; i++)
scanf("%d", &A[i]);
printf("\nReading array B (enter %d integer)\n", N);
for (i = 0; i < N; i++)
scanf("%d", &B[i]);
for (i = 0, j = N - 1; i < N; i++,j--)
C[i] = A[j] + B[i];
printf("\nPrinting array C\n");
for (i = 0; i < N; i++)
printf("%d ", C[i]);
}
Back to the list of exercises
Looking for a more challenging exercise, try this one !!
