Sort the elements of an array A in ascending order.
Method: Traverse the array from left to right using the index I. For each element A[I] of the array, determine the PMIN position of the (first) minimum to the right of A[I] and exchange A[I] and A[PMIN].
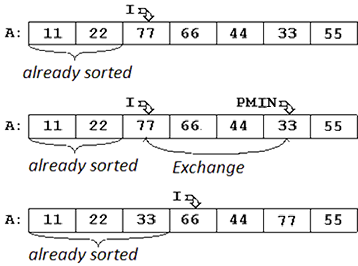
Difficulty level

Video recording
This exercise is mostly suitable for students
#include<stdio.h>
#include<conio.h>
#define SIZE 50
void main()
{
int A[SIZE], N;
int i, j, pmin, aux;
do {
printf("Enter the dimension: ");
scanf("%d", &N);
} while (N <= 0 || N > SIZE);
for (i = 0; i < N; i++)
{
printf("Enter A[%d]: ", i);
scanf("%d", &A[i]);
}
// nb of steps
for (i = 0; i < N - 1; i++)
{
pmin = i;
for (j = i + 1; j < N; j++)
if (A[j] < A[pmin])
pmin = j;
if (pmin != i)
{
aux = A[pmin];
A[pmin] = A[i];
A[i] = aux;
}
}
printf("\n\n** After sorting ** \n");
for (i = 0; i < N; i++)
{
printf("A[%d]=%d\n", i, A[i]);
}
getch();
}
Back to the list of exercises
Looking for a more challenging exercise, try this one !!
