Consider the following tree:
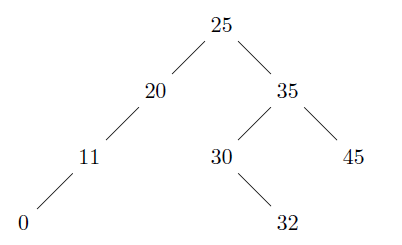
- Give the inorder traversal of the above tree.
- Write a recursive function that prints the inorder traversal of a tree dynamically implemented.
- Write an iterative function that prints the inorder traversal of a tree dynamically implemented.
Difficulty level

This exercise is mostly suitable for students
1. 0 - 11 - 20 - 25 - 30 - 32 - 35 - 45
2.
void InOrder(Btree B)
{
if(B)
{
InOrder(B->left);
printf("%d", B->data);
InOrder(B->right);
}
}
3.
void InOrderI(Btree B)
{
stack s = CreateStack();
if(!B) return;
while(1)
{
while(B)
{
Push(&s,B);
B=B->left;
}
if(Top(s,&B))
{
Pop(&s);
printf("%d ", B->data);
B=B->right;
}
else
break;
}
}
Back to the list of exercises
Looking for a more challenging exercise, try this one !!
